Do-while loop in C language
The condition is verified at the conclusion of the loop rather than at the beginning, which is a fundamental distinction between the C do-while loop and the while loop. This indicates that whether the condition is initially true or false, the body of the loop will always be run at least once.
Basic syntax of Do-while loop:
do {
// statements to be executed
} while (condition);
As long as the condition is true, the statements inside the loop will be repeated. Each time the loop iterates, the condition is verified. The loop continues if it is still true; otherwise, it ends.
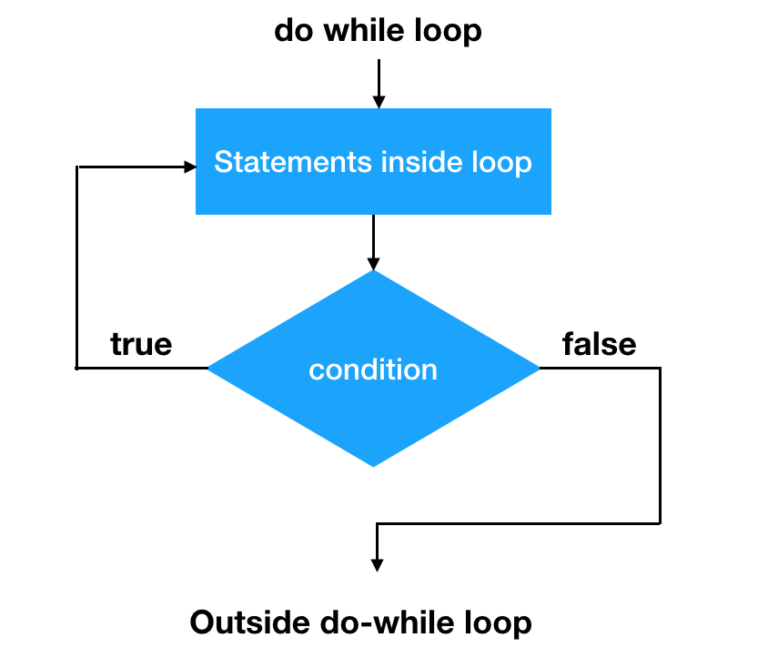
Example of Do-while loop:
#include <stdio.h>
int main() {
int num, sum = 0;
do {
printf("Enter a positive integer (enter 0 to end): ");
scanf("%d", &num);
sum += num;
} while (num != 0);
printf("The sum is %d\n", sum);
return 0;
}
Output:
Enter a positive integer (enter 0 to end): 5
Enter a positive integer (enter 0 to end): 2
Enter a positive integer (enter 0 to end): 8
Enter a positive integer (enter 0 to end): 0
The sum is 15
In this example, the loop continues to ask the user for positive integers and adds them to the sum variable until the user enters 0. The loop will always execute at least once because the condition is checked at the end of each iteration. Note that if the user enters 0 as the first input, the loop will terminate immediately, without adding anything to the sum.
Advantages of do-while loop:
It is advantageous to utilise the loop since it will always run at least once if you need to carry out an action before testing a condition.
The loop structure is simpler to read and comprehend than alternative loop forms, which reduces the likelihood of errors.
The loop body can be used to repeatedly ask the user for input, and the loop condition can be used to determine whether the input is legitimate.
Disadvantages of do-while loop:
If the condition is not properly defined or if the loop body does not update the loop condition, it may result in infinite loops.
While the loop body will always execute at least once, it can be less effective than other loop structures if the loop condition is known before entering the loop.
When the loop condition and the loop body are complex, it could be more challenging to maintain and modify than other loop structures.