For loop in C language
The for loop is a type of loop that allows you to repeat a block of code a fixed number of times.
Basic syntax of for loop:
for (initialization; condition; increment/decrement) {
statement(s);
}
- Initialization: It is an expression that is executed only once at the beginning of the loop. It is used to initialize the loop counter.
- Condition: It is an expression that is evaluated at the beginning of each iteration. If the condition is true, the loop body is executed, otherwise, the loop is terminated.
- Increment/Decrement: It is an expression that is executed at the end of each iteration. It is used to update the loop counter.
- statement(s): The statements inside the curly braces will be executed repeatedly as long as the condition is true.
Here, the initialization statement is executed once before the loop starts, the condition is checked before each iteration of the loop, and the increment/decrement statement is executed at the end of each iteration.
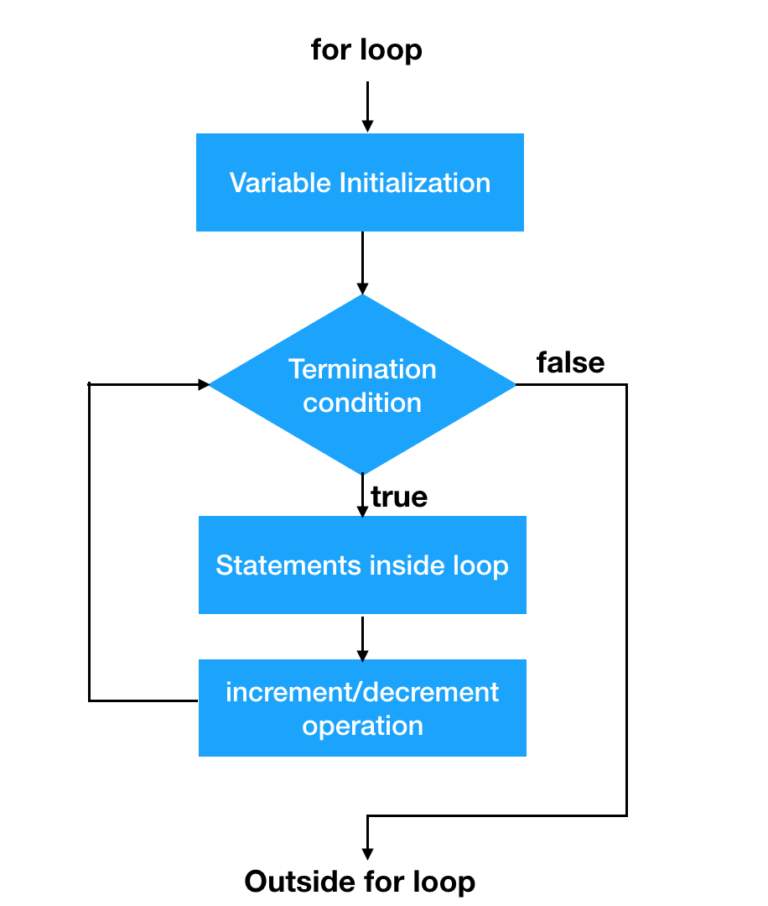
Example of for loop:
#include <stdio.h>
int main() {
int i;
for (i = 1; i <= 10; i++) {
printf("%d ", i);
}
return 0;
}
Output:
1 2 3 4 5 6 7 8 9 10
In this example, the loop control variable i is initialized to 1. The loop continues as long as i is less than or equal to 10. The loop body simply prints the value of i to the screen, followed by a space. At the end of each iteration, the value of i is incremented by 1. Therefore, the loop will print the numbers from 1 to 10.
Another example of for loop:
#include <stdio.h>
int main() {
int num, i, fact = 1;
printf("Enter a positive integer: ");
scanf("%d", &num);
// calculate factorial of num
for (i = 1; i <= num; i++) {
fact *= i;
}
printf("Factorial of %d is %d\n", num, fact);
return 0;
}
Output:
Enter a positive integer: 5
Factorial of 5 is 120
Here, we first take input from the user for the number whose factorial needs to be calculated. Then, we use a for loop to calculate the factorial of the number. The loop starts from 1 and iterates until the number itself, multiplying the value of fact by the loop variable i in each iteration. Finally, we print out the result.
Advantages of for loop:
The for loop can be used to run a piece of code a certain number of times.
For iterating over a range of values, it offers a straightforward and condensed syntax.
It enables more precise control over the loop’s variables and its iteration count.
It is quicker to iterate over a range of numbers than the while loop and do-while loop.
Disadvantages of for loop:
- When iterating through intricate data structures like linked lists or trees, it might be challenging.
- For some sorts of loops, including those that depend on user input to decide when to stop iterating, it could be less simple to use.
- When the number of iterations is uncertain or unexpected, it is not appropriate.