One-dimensional arrays in C language
A one-dimensional array in the C programming language is a group of identically typed elements kept in a single block of memory. An index that begins at 0 and ends at the array size minus one is used to access each element in the array.
Syntax for declaring a one-dimensional array:
datatype arrayName[arraySize];
Here, array name is the name of the array, array size is the maximum number of elements the array can carry, and data type is the type of data that the array will hold (such as int, float, char, etc.).
When we need to store and manage a lot of the same sort of data, arrays come in handy. For instance, we can define an array of size 100 instead of 100 variables if we need to hold the grades of 100 students.
Example of one-dimensional array:
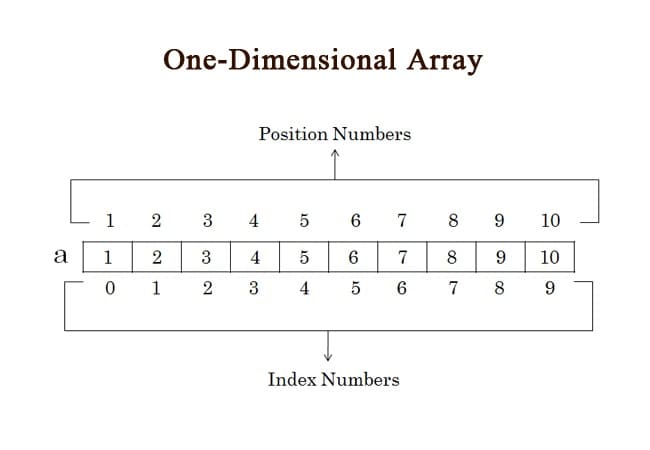
int marks[5]; // declares an array of 5 integers
Moreover, you can initialize the array when it is declared by including a list of values separated by commas and encased in curly braces, as seen in the following example:
int numbers[5] = {1, 2, 3, 4, 5};
This creates an array named numbers with 5 elements and initializes them with the values 1, 2, 3, 4, and 5.
You can access individual elements of the array using the index notation arrayName[index], where arrayName is the name of the array, and index is the index of the element you want to access. For example:
int x = numbers[2]; // assigns the value 3 to x
In this example, the third element of the numbers array is accessed using the index 2 and its value is assigned to the variable x.
Another example of one-dimensional array:
#include <stdio.h>
int main() {
int numbers[5] = {10, 20, 30, 40, 50};
printf("The first number in the array is %d\n", numbers[0]);
printf("The third number in the array is %d\n", numbers[2]);
printf("The last number in the array is %d\n", numbers[4]);
return 0;
}
Output:
The first number in the array is 10
The third number in the array is 30
The last number in the array is 50
This creates an integer array named numbers with five elements, initialized with the values 10, 20, 30, 40, and 50. The first element of the array can be accessed with numbers[0], the second with numbers[1], and so on. The program prints out the values of the first, third, and last elements of the array. The output would be: