While loop in C language
While a certain condition is true, you can continually run a block of code using the while loop control flow statement in C.
Basic syntax of while loop:
while (condition) {
// code to be executed
}
In the above syntax, condition is a Boolean expression that is evaluated before each iteration of the loop. If the condition is true, the code within the loop is executed. After the loop body is executed, the condition is evaluated again, and if it’s still true, the loop body is executed again, and this process repeats until the condition becomes false.
Example of while loop condition:
#include <stdio.h>
int main() {
int num = 10;
while (num >= 1) {
printf("%d ", num);
num--;
}
printf("\nDone!");
return 0;
}
Output:
1 2 3 4 5 6 7 8 9 10
In the above example, the while loop iterates as long as the value of i is less than or equal to 10. During each iteration, the value of i is printed to the console, and then i is incremented by 1. The loop continues until i is no longer less than or equal to 10.
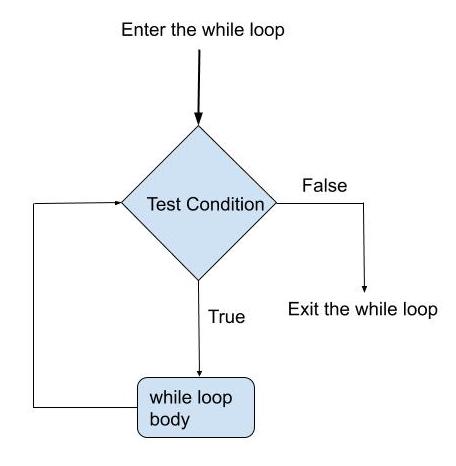
Another example of while loop:
#include <stdio.h>
int main() {
int num = 10;
while (num >= 1) {
printf("%d ", num);
num--;
}
printf("\nDone!");
return 0;
}
Output:
10 9 8 7 6 5 4 3 2 1
Done!
In this example, the loop starts with num set to 10, and the loop will continue as long as num is greater than or equal to 1. Inside the loop, the current value of num is printed on the screen, and then num is decremented by 1. This process repeats until num is no longer greater than or equal to 1. Finally, the message “Done!” is printed to indicate that the loop has finished. The output of this program would be:
Advantages of while loop:
It is simple to comprehend and use.
When the precise number of iterations is unknown beforehand, it is helpful.
If a condition is met, it can be used to repeat a block of code.
Disadvantages of while loop:
If the condition is never false, it can result in an indefinite loop.
If the condition is false right away, it might never execute.
The loop control variable may need to be initialized and updated with additional code.