C++ Do-while loop
Do-while loops are control flow statements in C++ that repeatedly run a block of code until the desired condition is false. A do-while loop always runs the code block at least once, unlike the while loop which only does so when the condition is first true.
Basic syntax of Do-while loop:
do {
// code to be executed
} while (condition);
Here, until the condition inside the parenthesis () is true, the code inside the curly braces is continually performed. At the conclusion of each iteration, the condition is verified; if true, the loop continues to run. If the predicate is false, the loop ends, and the statement after the loop is executed next in the program.
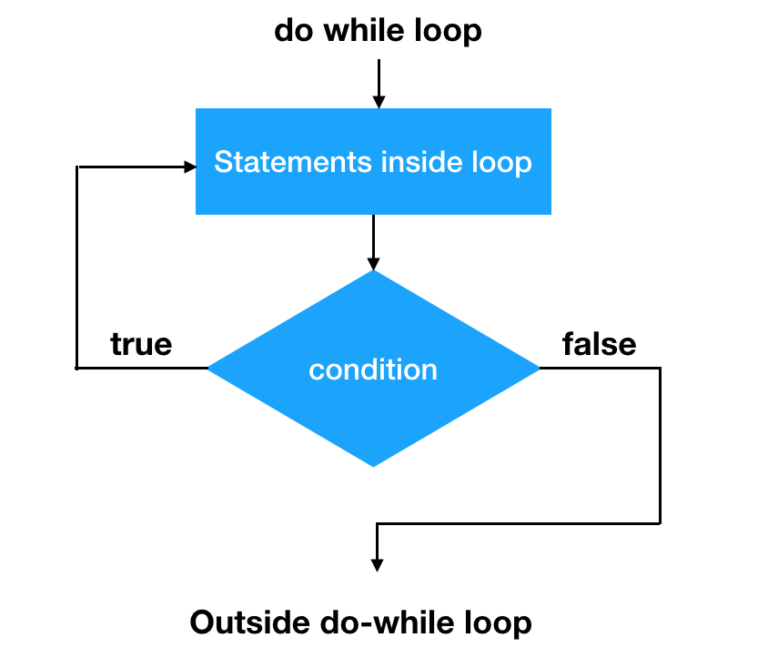
Example of Do-while loop:
#include <iostream>
using namespace std;
int main() {
int i = 1;
do {
cout << i << " ";
i++;
} while (i <= 10);
return 0;
}
Output:
1 2 3 4 5 6 7 8 9 10
Another Example:
#include <iostream>
#include <cstdlib>
using namespace std;
int main() {
int num;
char choice;
do {
num = rand() % 100 + 1; // generate a random number between 1 and 100
cout << "The random number is: " << num << endl;
cout << "Do you want to generate another random number? (y/n): ";
cin >> choice;
} while (choice == 'y' || choice == 'Y');
return 0;
}
Output:
The random number is: 42
Do you want to generate another random number? (y/n): y
The random number is: 87
Do you want to generate another random number? (y/n): y
The random number is: 3
Do you want to generate another random number? (y/n): n
Advantages of do-while loop:
It is advantageous to utilize the loop since it will always run at least once if you need to carry out an action before testing a condition.
The loop structure is simpler to read and comprehend than alternative loop forms, which reduces the likelihood of errors.
The loop body can be used to repeatedly ask the user for input, and the loop condition can be used to determine whether the input is legitimate.
Disadvantages of do-while loop:
If the condition is not properly defined or if the loop body does not update the loop condition, it may result in infinite loops.
While the loop body will always execute at least once, it can be less effective than other loop structures if the loop condition is known before entering the loop.
When the loop condition and the loop body are complex, it could be more challenging to maintain and modify than other loop structures.