C++ While loop
The while loop is a control flow statement in C++ that enables you to repeatedly run a block of code so long as a predetermined condition is true.
Basic syntax of while loop:
while (condition) {
// Code block to be executed repeatedly
}
Each time the loop iterates, the condition is checked to see if it is true. If it is, the code block inside the loop is then run. If the predicate is false, the loop ends and the statement following the loop receives program control.
Example of while loop condition:
#include <iostream>
int main() {
int i = 1;
while (i <= 5) {
std::cout << i << "\n";
i++;
}
return 0;
}
Output:
1
2
3
4
5
In this instance, the loop runs for as long as the variable “i” has a value that is less than or equal to 5. Simply printing the value of “i” to the console, followed by a newline character, is all that the code block inside the loop does. The value of “i” is raised by 1 at the conclusion of each iteration.
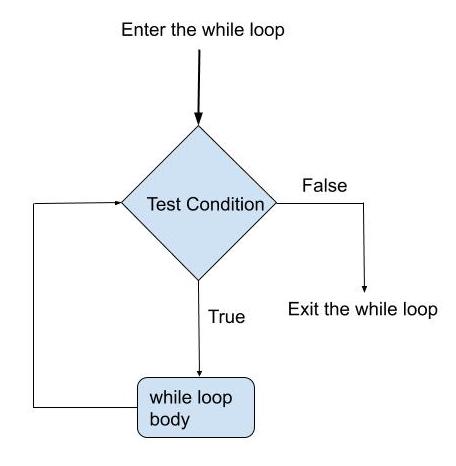
Another example of while loop:
#include <iostream>
int main() {
int i = 1;
int sum = 0;
while (i <= 10) {
sum += i;
i++;
}
std::cout << "The sum of the first 10 natural numbers is: " << sum << "\n";
return 0;
}
Output:
The sum of the first 10 natural numbers is: 55
In this illustration, the loop runs as long as the variable “i”‘s value is less than or equal to 10. The code block that makes up the loop calculates and stores the sum of the first “i” natural numbers. The value of “i” is raised by 1 at the conclusion of each iteration. The program writes the value of “sum” to the console after the loop ends.
Advantages of while loop:
It is simple to comprehend and use.
When the precise number of iterations is unknown beforehand, it is helpful.
If a condition is met, it can be used to repeat a block of code.
Disadvantages of while loop:
If the condition is never false, it can result in an indefinite loop.
If the condition is false right away, it might never execute.
The loop control variable may need to be initialized and updated with additional code.